Top 50 JavaScript coding Interview Questions and Answers in 2024
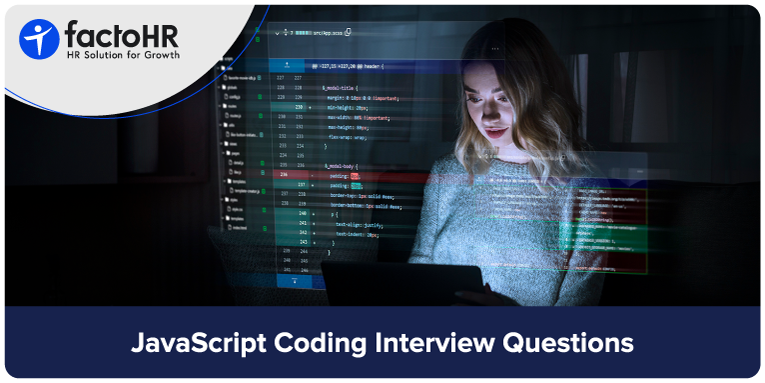
Table of Contents
JavaScript is a highly versatile programming language widely employed for creating interactive and dynamic web pages. It serves as the primary language for front-end developers working on web applications. Additionally, JavaScript is utilized in back-end development, allowing for full-stack application creation. The language is supported by various frameworks, such as jQuery, ReactJS, and AngularJS, which enhance its capabilities and ease of use. Given its popularity and complexity, interviews focused on JavaScript tend to be quite challenging for candidates.
This guide provides a complete overview of JavaScript coding interview questions. These top 50 JavaScript coding interview questions and answers will help anyone thoroughly practice and prepare for the interview. This guide advises on coding challenges for beginners, mid-level, and expert JavaScript developers.
JavaScript Coding Interview Questions and Answers
Basic JavaScript Coding Interview Questions For Fresher
Basic JavaScript coding includes variables, operators, control flows, functions, arrays, DOM manipulation (document object model), and many more. The basic JavaScript coding questions are mentioned below.
1. Write a JavaScript function that calculates the sum of two numbers
Answer
function sum(a, b) { return a + b; } const num1 = 10; const num2 = 20; const result = sum(num1, num2); console.log("The sum of", num1, "and", num2, "is", result);
2. Write a JavaScript program to reverse the given string
Answer
function reverseString(str) { let reversed = ""; for (let i = str.length - 1; i >= 0; i--) { reversed += str[i]; } return reversed; } const inputString = "hello world"; const reversedString = reverseString(inputString); console.log("Reversed string:", reversedString);
3. Write a JavaScript function that finds the maximum number in an array
Answer
function findMax(arr) { if (arr.length === 0) { return null; // Handle empty array } let max = arr[0]; for (let i = 1; i < arr.length; i++) { if (arr[i] > max) { max = arr[i]; } } return max; } const numbers = [10, 5, 25, 8, 15]; const maxNumber = findMax(numbers); console.log("The maximum number is:", maxNumber);
4. Write a JavaScript program that calculates the factorial of a number
Answer
function factorial(n) { if (n === 0 || n === 1) { return 1; } else { return n * factorial(n - 1); } } const number = 5; const result = factorial(number); console.log("Factorial of", number, "is", result);
5. Write a JavaScript program to check whether the given number is prime
Answer
function isPrime(number) { if (number <= 1) { return false; // Numbers less than or equal to 1 are not prime } for (let i = 2; i * i <= number; i++) { if (number % i === 0) { return false; // If divisible by any number between 2 and the square root of a number, it's not prime } } return true; // If the loop completes without finding a divisor, the number is prime } const num = 17; const isPrimeResult = isPrime(num); if (isPrimeResult) { console.log(num, "is a prime number."); } else { console.log(num, "is not a prime number."); }
6. Write a JavaScript function that uses an array of numbers and only gives a new array with even numbers
Answer
function evenNumbers(numbers) { const evenArray = []; for (let i = 0; i < numbers.length; i++) { if (numbers[i] % 2 === 0) { evenArray.push(numbers[i]); } } return evenArray; } const numbers = [1, 2, 3, 4, 5, 6, 7, 8]; const evenNumbersArray = evenNumbers(numbers); console.log("Even numbers:", evenNumbersArray);
7. Write a JavaScript program to change the string to title (first letter of every word should be in upper case)
Answer
function capitalizeFirstLetterOfEachWord(str) { const words = str.split(" "); const capitalizedWords = words.map(word => { return word.charAt(0).toUpperCase() + word.slice(1); }); return capitalizedWords.join(" "); } const inputString = "hello world this is a sample string"; const capitalizedString = capitalizeFirstLetterOfEachWord(inputString); console.log("Capitalized string:", capitalizedString);
8. Write a JavaScript function to find the largest element in the nested array
Answer
function findLargestElement(arr) { let max = -Infinity; function helper(nestedArr) { for (let item of nestedArr) { if (typeof item === "number") { max = Math.max(max, item); } else if (Array.isArray(item)) { helper(item); } } } helper(arr); return max; } const nestedArray = [1, [2, 3, [4, 5]], 6, [7, 8]]; const largestElement = findLargestElement(nestedArray); console.log("Largest element:", largestElement);
9. Write a JavaScript program to check whether the given string is a palindrome. (that reads the same from forward and backward)
Answer
function isPalindrome(str) { const reversedStr = str.split("").reverse().join(""); return str === reversedStr; } const inputString = "racecar"; const isPalindromeResult = isPalindrome(inputString); if (isPalindromeResult) { console.log(inputString, "is a palindrome."); } else { console.log(inputString, "is not a palindrome."); }
10. Write a JavaScript function that returns the Fibonacci sequence to a specified number
Answer
function fibonacci(n) { if (n <= 0) { return []; } if (n === 1) { return [0]; // Return [0] if n is 1 } const sequence = [0, 1]; for (let i = 2; i < n; i++) { sequence.push(sequence[i - 1] + sequence[i - 2]); } return sequence; } const terms = 10; const fibonacciSequence = fibonacci(terms); console.log(fibonacciSequence);
Advanced JavaScript Coding Interview Questions
Advanced JavaScript coding questions includes certain tricky and complex concepts and can only be practiced after gaining fundamental knowledge of basic JS coding. After having a concrete understanding, one can enhance their skills and create more complex applications. Some of these concepts are practically tested in an interview to evaluate the interviewee. This includes asynchronous programming, Object-oriented programming (OOP), closures, ES6+ features, and many more. The advanced JavaScript coding interview questions are mentioned here.
1. Write a repetitive function to find a factorial of a given number
Answer
function factorial(n) { if (n === 0 || n === 1) { return 1; } else { return n * factorial(n - 1); } } const number = 5; const result = factorial(number); console.log("Factorial of " + number + " is " + result);
2. Develop a single-level array by functioning it into a flat nested array
Answer
function flattenArray(arr) { const flattened = []; function flattenHelper(nestedArr) { for (let item of nestedArr) { if (Array.isArray(item)) { flattenHelper(item); // Recursively flatten the nested array } else { flattened.push(item); // Push non-array items into the flattened array } } } flattenHelper(arr); return flattened; } const nestedArray = [1, [2, 3], 4, [5, 6, [7, 8]]]; const flattenedArray = flattenArray(nestedArray); console.log("Flattened array:", flattenedArray);
3. Make a debounce function in JavaScript that limits the occurrence of a function’s execution
Answer
function debounce(func, delay) { let timeoutId; return function (...args) { clearTimeout(timeoutId); timeoutId = setTimeout(() => { func.apply(this, args); }, delay); }; } // Example usage: function handleInputChange(event) { console.log(event.target.value); } // Debounce with a 500ms delay const debouncedHandleInputChange = debounce(handleInputChange, 500); // Ensure the DOM is fully loaded before attaching the event listener document.addEventListener("DOMContentLoaded", () => { const inputElement = document.getElementById("myInput"); if (inputElement) { inputElement.addEventListener("input", debouncedHandleInputChange); } else { console.error("Element with ID 'myInput' not found."); } });
4. Write a JavaScript function that directs whether the given strings are anagrams
Answer
function areAnagrams(str1, str2) { // Check if the strings have different lengths if (str1.length !== str2.length) { return false; } // Create character count objects for both strings const charCount1 = {}; const charCount2 = {}; // Count characters in the first string for (let char of str1) { charCount1[char] = (charCount1[char] || 0) + 1; } // Count characters in the second string for (let char of str2) { charCount2[char] = (charCount2[char] || 0) + 1; } // Compare character counts for (let char in charCount1) { if (charCount1[char] !== charCount2[char]) { return false; } } return true; } // Example usage: const str1 = "anagram"; const str2 = "nagaram"; if (areAnagrams(str1, str2)) { console.log("The strings are anagrams."); } else { console.log("The strings are not anagrams."); }
5. Write a JavaScript function that uses an array of objects and keys and shows a new array based on the values of the key in ascending order
Answer
function sortByKeyValue(arr, key) { return arr.sort((a, b) => { if (a[key] < b[key]) { return -1; } else if (a[key] > b[key]) { return 1; } else { return 0; } }); } const data = [ { name: "Alice", age: 25 }, // Removed the comma after "Alice" { name: "Bob", age: 30 }, { name: "Charlie", age: 20 } ]; const sortedData = sortByKeyValue(data, "age"); console.log(sortedData);
6. Make a function without any built-in sort functions that use two sorted arrays and merge them into one array
Answer
function mergeSortedArrays(arr1, arr2) { let merged = []; let i = 0; let j = 0; while (i < arr1.length && j < arr2.length) { if (arr1[i] < arr2[j]) { merged.push(arr1[i]); i++; } else { merged.push(arr2[j]); j++; } } // Append remaining elements from arr1 while (i < arr1.length) { merged.push(arr1[i]); i++; } // Append remaining elements from arr2 while (j < arr2.length) { merged.push(arr2[j]); j++; } return merged; } const arr1 = [1, 3, 5, 7]; const arr2 = [2, 4, 6, 8]; const mergedArray = mergeSortedArrays(arr1, arr2); console.log(mergedArray); // Output: [1, 2, 3, 4, 5, 6, 7, 8]
7. Make a deep clone function in JavaScript that makes an array or a nested object without any original reference
Answer
function deepClone(obj) { if (typeof obj !== "object" || obj === null) { return obj; // Handle primitive values and null } const clone = Array.isArray(obj) ? [] : {}; for (const key in obj) { if (obj.hasOwnProperty(key)) { clone[key] = deepClone(obj[key]); } } return clone; } // Example usage: const originalObject = { // Fixed variable name by removing space name: "Alice", // Added missing comma here age: 30, address: { street: "123 Main St", city: "Anytown" }, numbers: [1, 2, 3] }; const clonedObject = deepClone(originalObject); // Modify the cloned object clonedObject.age = 31; clonedObject.address.city = "Newtown"; clonedObject.numbers.push(4); console.log("Original object:", originalObject); console.log("Cloned object:", clonedObject);
8. Write a JavaScript function for a linked list that can add, remove, or insert nodes at the start, end, or specified position
Answer
class Node { constructor(data) { this.data = data; this.next = null; } } class LinkedList { constructor() { this.head = null; this.size = 0; } // Insert a node at the beginning insertAtBeginning(data) { const newNode = new Node(data); newNode.next = this.head; this.head = newNode; this.size++; } // Insert a node at the end insertAtEnd(data) { const newNode = new Node(data); if (!this.head) { this.head = newNode; } else { let current = this.head; while (current.next) { current = current.next; } current.next = newNode; } this.size++; } // Insert a node at a specific position insertAt(data, index) { if (index < 0 || index > this.size) { return; // Invalid index } if (index === 0) { this.insertAtBeginning(data); } else if (index === this.size) { this.insertAtEnd(data); } else { const newNode = new Node(data); let current = this.head; let previous = null; let i = 0; while (i < index) { previous = current; current = current.next; i++; } newNode.next = current; previous.next = newNode; } this.size++; } // Remove a node at a specific position deleteAt(index) { if (index < 0 || index >= this.size) { return; // Invalid index } if (index === 0) { this.head = this.head.next; } else { let current = this.head; let previous = null; let i = 0; while (i < index) { previous = current; current = current.next; i++; } previous.next = current.next; } this.size--; } // Traverse the linked list and print the data printList() { let current = this.head; while (current) { console.log(current.data); current = current.next; } } } // Example usage: const linkedList = new LinkedList(); linkedList.insertAtBeginning(10); linkedList.insertAtEnd(20); linkedList.insertAt(30, 1); linkedList.printList(); // Output: 10 30 20 linkedList.deleteAt(1); linkedList.printList(); // Output: 10 20
9. Write a JavaScript function using memorization for optimal efficiency where the number returns the Fibonacci sequence up to a specified number
Answer
function fibonacciMemoized(n, memo = {}) { if (n <= 0) { return 0; // This handles the case for 0 and negative inputs } if (n === 1 || n === 2) { return 1; // Base cases for Fibonacci } if (memo[n] !== undefined) { return memo[n]; // Return cached result if it exists } const result = fibonacciMemoized(n - 1, memo) + fibonacciMemoized(n - 2, memo); memo[n] = result; // Cache the result for future use return result; } const terms = 10; const fibonacciSequence = []; for (let i = 1; i <= terms; i++) { // Start from 1 to generate the first 10 Fibonacci numbers fibonacciSequence.push(fibonacciMemoized(i)); } console.log(fibonacciSequence);
10. Consider only alphanumeric characters and ignore the case. Develop a program that checks if the given string is a palindrome
Answer
function isPalindrome(str) { // Remove non-alphanumeric characters and convert to lowercase const cleanedStr = str.replace(/[^a-zA-Z0-9]/g, '').toLowerCase(); // Check if the cleaned string equals its reversed version return cleanedStr === cleanedStr.split('').reverse().join(''); } // Example usage: const input1 = "A man, a plan, a canal: Panama"; const input2 = "race a car"; console.log(isPalindrome(input1)); // Output: true console.log(isPalindrome(input2)); // Output: false
Common JavaScript Coding Interview Questions
Some common JavaScript coding questions are asked to ensure the interviewee knows certain underlying functions, such as algorithms, debugging and problem-solving, prototype inheritance, etc.
1. Write a program that finds the sum of all the numbers in an array
Answer
function sumOfNumbers(arr) { let sum = 0; for (let i = 0; i < arr.length; i++) { sum += arr[i]; } return sum; } const numbers = [1, 2, 3, 4, 5]; const result = sumOfNumbers(numbers); console.log("The sum of the numbers is:", result);
2. Write a function that finds the factorial of a given number
Answer
function factorial(n) { if (n === 0 || n === 1) { return 1; // Base case: factorial of 0 or 1 is 1 } else { return n * factorial(n - 1); // Recursive case } } const number = 5; // Example number const result = factorial(number); // Calculate factorial console.log("Factorial of " + number + " is " + result); // Output the result
3. Write a JavaScript program to check whether the given number is prime
Answer
function isPrime(number) { if (number <= 1) { return false; // Numbers less than or equal to 1 are not prime } for (let i = 2; i * i <= number; i++) { if (number % i === 0) { return false; // If divisible by any number between 2 and the square root of the number, it's not prime } } return true; // If the loop completes without finding a divisor, the number is prime } const num = 17; // Example number to check const isPrimeResult = isPrime(num); // Check if the number is prime if (isPrimeResult) { console.log(num + " is a prime number."); // Output if the number is prime } else { console.log(num + " is not a prime number."); // Output if the number is not prime }
4. Write a program to check whether the given string is a palindrome
Answer
function isPalindrome(str) { // Remove non-alphanumeric characters and convert to lowercase const cleanedStr = str.replace(/[^a-zA-Z0-9]/g, '').toLowerCase(); // Check if the cleaned string equals its reversed version return cleanedStr === cleanedStr.split('').reverse().join(''); } // Example usage: const input1 = "A man, a plan, a canal: Panama"; const input2 = "race a car"; console.log(isPalindrome(input1)); // Output: true console.log(isPalindrome(input2)); // Output: false
5. Applying the function to reverse a string without using the built-in reverse method
Answer
function reverseString(str) { let reversed = ""; for (let i = str.length - 1; i >= 0; i--) { reversed += str[i]; } return reversed; } const inputString = "hello world"; const reversedString = reverseString(inputString); console.log("Reversed string:", reversedString);
6. Write a function that removes the duplicates from an array
Answer
function removeDuplicates(arr) { const uniqueSet = new Set(arr); return Array.from(uniqueSet); } const numbers = [1, 2, 3, 2, 4, 5, 1, 4]; const uniqueNumbers = removeDuplicates(numbers); console.log("Unique numbers:", uniqueNumbers);
7. Write a function that counts the occurrences of each character in the given string
Answer
function countCharacterOccurrences(str) { const charCount = {}; for (let char of str) { charCount[char] = (charCount[char] || 0) + 1; } return charCount; } const inputString = "hello world"; const characterCounts = countCharacterOccurrences(inputString); console.log("Character counts:", characterCounts);
8. Write a function that categorizes an array of numbers in ascending order
Answer
function sortNumbersAscending(arr) { return arr.sort((a, b) => a - b); } const numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5]; const sortedNumbers = sortNumbersAscending(numbers); console.log(sortedNumbers); // Output: [1, 1, 2, 3, 4, 5, 5, 6, 9]
9. Write a function to find the largest and smallest numbers in the array from the set of a given array of numbers
Answer
function findLargestAndSmallest(arr) { let largest = arr[0]; let smallest = arr[0]; for (let i = 1; i < arr.length; i++) { if (arr[i] > largest) { largest = arr[i]; } else if (arr[i] < smallest) { smallest = arr[i]; } } return { largest, smallest }; } const numbers = [10, 5, 25, 8, 15]; const result = findLargestAndSmallest(numbers); console.log("Largest number:", result.largest); console.log("Smallest number:", result.smallest);
10. Write a function that takes an array of numbers as input and returns a new array with unique elements only
Answer
function findLargestAndSmallest(arr) { let largest = arr[0]; let smallest = arr[0]; for (let i = 1; i < arr.length; i++) { if (arr[i] > largest) { largest = arr[i]; } else if (arr[i] < smallest) { smallest = arr[i]; } } return { largest, smallest }; } const numbers = [10, 5, 25, 8, 15]; const result = findLargestAndSmallest(numbers); console.log("Largest number:", result.largest); console.log("Smallest number:", result.smallest);
Tricky JavaScript Coding Interview Questions
This section of JavaScript coding interview questions is challenging as the questions get trickier. Still, it also shows the ability to understand the concepts of advanced JavaScript and problem-solving abilities. This includes carrying, event delegation, expressions, design patterns, etc.
1. Write a function that takes a number and returns with its factorial
Answer
function factorial(n) { if (n === 0 || n === 1) { return 1; } else { return n * factorial(n - 1); } } const number = 5; const result = factorial(number); console.log("Factorial of " + number + " is " + result);
2. Write a function that flattens a nested array in a single-dimensional array
Answer
function flattenArray(arr) { const flattened = []; function flattenHelper(nestedArr) { for (let item of nestedArr) { if (Array.isArray(item)) { flattenHelper(item); // Recursively flatten nested arrays } else { flattened.push(item); // Add non-array items to the flattened array } } } flattenHelper(arr); return flattened; } const nestedArray = [1, [2, 3], 4, [5, 6, [7, 8]]]; const flattenedArray = flattenArray(nestedArray); console.log("Flattened array:", flattenedArray); // Output: [1, 2, 3, 4, 5, 6, 7, 8]
3. Write a function that finds the second element in an array of integers
Answer
function findSecondElement(arr) { if (arr.length >= 2) { return arr[1]; } else { return null; // Handle case when the array has less than 2 elements } } const numbers = [1, 2, 3, 4, 5]; const secondElement = findSecondElement(numbers); console.log("Second element:", secondElement);
4. Write a function that verifies that the given string is a palindrome while excluding whitespaces and punctuations
Answer
function isPalindrome(str) { // Remove non-alphanumeric characters and convert to lowercase const cleanedStr = str.replace(/[^a-zA-Z0-9]/g, '').toLowerCase(); // Check if the cleaned string equals its reversed version return cleanedStr === cleanedStr.split('').reverse().join(''); } // Example usage: const input1 = "A man, a plan, a canal: Panama"; const input2 = "race a car"; console.log(isPalindrome(input1)); // Output: true console.log(isPalindrome(input2)); // Output: false
5. Write a function that keeps only the unique elements while removing the duplicate from an array
Answer
function removeDuplicates(arr) { const uniqueSet = new Set(arr); return Array.from(uniqueSet); } const numbers = [1, 2, 3, 2, 4, 5, 1, 4]; const uniqueNumbers = removeDuplicates(numbers); console.log("Unique numbers:", uniqueNumbers);
6. Write a function that checks whether the given string is an anagram of another string
Answer
function areAnagrams(str1, str2) { // Check if the strings have different lengths if (str1.length !== str2.length) { return false; } // Create character count objects for both strings const charCount1 = {}; const charCount2 = {}; // Count characters in the first string for (let char of str1) { charCount1[char] = (charCount1[char] || 0) + 1; } // Count characters in the second string for (let char of str2) { charCount2[char] = (charCount2[char] || 0) + 1; } // Compare character counts for (let char in charCount1) { if (charCount1[char] !== charCount2[char]) { return false; } } return true; } // Example usage: const str1 = "anagram"; const str2 = "nagaram"; if (areAnagrams(str1, str2)) { console.log("The strings are anagrams."); } else { console.log("The strings are not anagrams."); }
7. Write a function that generates a random alphanumeric string of a specified length
Answer
function generateRandomString(length) { const characters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789'; let randomString = ''; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * characters.length); randomString += characters.charAt(randomIndex); } return randomString; } const randomString = generateRandomString(10); console.log(randomString);
8. Without using the built-in reverse functionality, write a program that reverses the order of words in a sentence
Answer
function reverseWords(sentence) { const words = sentence.split(" "); const reversedWords = []; for (let i = words.length - 1; i >= 0; i--) { reversedWords.push(words[i]); } return reversedWords.join(" "); } const inputSentence = "Hello world this is a sample sentence"; const reversedSentence = reverseWords(inputSentence); console.log("Reversed sentence:", reversedSentence);
9. Write a program that uses the array of integers, resulting in the most significant difference between any two numbers
Answer
function findLargestDifference(arr) { let largestDifference = 0; for (let i = 0; i < arr.length - 1; i++) { for (let j = i + 1; j < arr.length; j++) { const currentDifference = Math.abs(arr[i] - arr[j]); if (currentDifference > largestDifference) { largestDifference = currentDifference; } } } return largestDifference; } const numbers = [10, 5, 25, 8, 15]; const largestDifferenceResult = findLargestDifference(numbers); console.log("Largest difference:", largestDifferenceResult);
10. Execute a function that converts a number into its Roman numerical representation
Answer
function convertToRoman(num) { const romanNumerals = [ { value: 1000, symbol: 'M' }, { value: 900, symbol: 'CM' }, { value: 500, symbol: 'D' }, { value: 400, symbol: 'CD' }, { value: 100, symbol: 'C' }, { value: 90, symbol: 'XC' }, { value: 50, symbol: 'L' }, { value: 40, symbol: 'XL' }, { value: 10, symbol: 'X' }, { value: 9, symbol: 'IX' }, { value: 5, symbol: 'V' }, { value: 4, symbol: 'IV' }, { value: 1, symbol: 'I' } ]; let roman = ''; for (let i = 0; i < romanNumerals.length; i++) { const numeral = romanNumerals[i]; while (num >= numeral.value) { roman += numeral.symbol; num -= numeral.value; } } return roman; } const number = 1234; const romanNumeral = convertToRoman(number); console.log("Roman numeral:", romanNumeral);
JavaScript Array Coding Questions
Arrays are fundamental data structures in JavaScript that have a collection of elements like array operations, manipulation, and specific advanced topics like depth-first search (DFS) or breadth-first search (BFS) algorithm, etc. One of the main reasons to ask array-related questions is to check a person’s ability to work with arrays and familiarity with fundamental data structures. Some of them are mentioned below.
1. Execute a function that shows the sum of all numbers in an array
Answer
function sumOfNumbers(arr) { let sum = 0; for (let i = 0; i < arr.length; i++) { sum += arr[i]; } return sum; } const numbers = [1, 2, 3, 4, 5]; const result = sumOfNumbers(numbers); console.log("The sum of the numbers is:", result);
2. Write a JavaScript function that shows an array's average value of numbers
Answer
function calculateAverage(arr) { if (arr.length === 0) { return 0; // Handle empty array } let sum = 0; for (let i = 0; i < arr.length; i++) { sum += arr[i]; } const average = sum / arr.length; return average; } const numbers = [10, 20, 30, 40, 50]; const averageValue = calculateAverage(numbers); console.log("The average value is:", averageValue);
3. Find a function that finds the maximum number in an array
Answer
function findMax(arr) { if (arr.length === 0) { return null; // Handle empty array } let max = arr[0]; for (let i = 1; i < arr.length; i++) { if (arr[i] > max) { max = arr[i]; } } return max; } const numbers = [10, 5, 25, 8, 15]; const maxNumber = findMax(numbers); console.log("The maximum number is:", maxNumber);
4. Write a JavaScript function that bifurcates the strings in alphabetical order
Answer
function bifurcateStrings(strings) { const firstHalf = []; const secondHalf = []; // Calculate the middle index const midIndex = Math.floor(strings.length / 2); // Get the reference string for comparison const referenceString = strings[midIndex]; for (const str of strings) { if (str.localeCompare(referenceString) <= 0) { firstHalf.push(str); } else { secondHalf.push(str); } } return [firstHalf, secondHalf]; } const strings = ["apple", "banana", "cherry", "date", "elderberry", "fig", "grape"]; const bifurcatedStrings = bifurcateStrings(strings); console.log(bifurcatedStrings);
5. Write a JavaScript function that shows a new array containing only the unique elements from an input array
Answer
function removeDuplicates(arr) { const uniqueSet = new Set(arr); return Array.from(uniqueSet); } const numbers = [1, 2, 3, 2, 4, 5, 1, 4]; const uniqueNumbers = removeDuplicates(numbers); console.log("Unique numbers:", uniqueNumbers);
6. Find the second largest number in an array and perform the function
Answer
function findSecondLargest(arr) { if (arr.length < 2) { return null; // Handle arrays with fewer than 2 elements } let firstLargest = Number.NEGATIVE_INFINITY; // Initialize to a very low number let secondLargest = Number.NEGATIVE_INFINITY; // Initialize to a very low number for (let i = 0; i < arr.length; i++) { if (arr[i] > firstLargest) { secondLargest = firstLargest; // Update second largest firstLargest = arr[i]; // Update first largest } else if (arr[i] > secondLargest && arr[i] < firstLargest) { secondLargest = arr[i]; // Update second largest only if it's less than the first largest } } return secondLargest === Number.NEGATIVE_INFINITY ? null : secondLargest; // Handle case where no second largest found } const numbers = [10, 5, 25, 8, 15]; const secondLargestNumber = findSecondLargest(numbers); console.log("Second largest number:", secondLargestNumber);
7. Write a JavaScript function by alternating the elements from each array and merging the two arrays into a single array
Answer
function mergeAlternatingElements(arr1, arr2) { const mergedArray = []; const maxLength = Math.max(arr1.length, arr2.length); for (let i = 0; i < maxLength; i++) { if (i < arr1.length) { mergedArray.push(arr1[i]); } if (i < arr2.length) { mergedArray.push(arr2[i]); } } return mergedArray; } const array1 = [1, 2, 3]; const array2 = [4, 5, 6, 7]; const mergedArray = mergeAlternatingElements(array1, array2); console.log(mergedArray); // Output: [1, 4, 2, 5, 3, 6, 7]
8. Write a JavaScript function that removes all the false values (false, null, 0, “”, unidentified, NaN) from an array
Answer
function removeFalseValues(arr) { return arr.filter(Boolean); // Filter out false values using Boolean as the filter function } const inputArray = [1, 0, false, null, "", "hello", undefined, NaN, 2]; // Fixed the string "hello" const filteredArray = removeFalseValues(inputArray); console.log("Filtered array:", filteredArray);
9. Create a function that groups elements in an array on a given condition. For example, arranging odd and even numbers in separate ways
Answer
function groupElements(arr, condition) { const group1 = []; const group2 = []; for (let element of arr) { if (condition(element)) { group1.push(element); } else { group2.push(element); } } return [group1, group2]; } // Example usage: const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]; // Group odd and even numbers const groupedNumbers = groupElements(numbers, (element) => element % 2 === 0); console.log("Group 1:", groupedNumbers[0]); console.log("Group 2:", groupedNumbers[1]);
10. Execute a function that finds the index of a specific element in an array, wherein if the element is not found, the function should return to -1
Answer
function findIndexOfElement(arr, element) { for (let i = 0; i < arr.length; i++) { if (arr[i] === element) { return i; } } return -1; } const numbers = [10, 5, 25, 8, 15]; const targetElement = 25; const index = findIndexOfElement(numbers, targetElement); if (index !== -1) { console.log("Element", targetElement, "found at index:", index); } else { console.log("Element", targetElement, "not found in the array."); }
Some Preparation Tips For JavaScript Interview
Here are some tips for preparing for a JavaScript coding interview.
1. Master The Fundamentals
Gain a perfect knowledge of JavaScript fundamentals and core concepts like functions, objects, data types, arrays, control flow, DOM manipulation, etc. Practice syntax, write clean and precise codes, and pay attention to the basic concepts.
2. Understand The Data Structures And Algorithms
Common data structures like arrays, queues, linked lists, stacks, trees, graphs, and many more should be covered and be familiar with the same. Algorithms like sorting (like bubble, merge, quick), searching (like linear, binary), and graph traversal practice and implement the same.
3. Practice The Coding
This is definitely one of the most essential tips to follow, as one must practice coding to pass an interview. Use platforms like LeetCode, HackeRanked, Codewars, and many more to solve coding issues in JavaScript. Practice coding questions under time constraints to develop time management skills as well. To do this, prepare your timesheet management software for a precise timeline.
4. Learn Common JavaScript Patterns
Some commonly used patterns are factory, singleton, observer, and module patterns, which should be understood and practiced. Get accustomed to the best practices for writing clean and efficient JavaScript code.
5. Stay Updated
To stay updated with the programming language, learn its modern features and best practices. Get accustomed to JavaScript frameworks and liberties like React, Angular, Vue.js, and many more.
By practicing and following these tips, anyone can boost their confidence and improve their chances of being selected for the JavaScript coding interview.
Wake-up Calls
The JavaScript coding interview consists of specific wake-up calls or red flags, as the interviewers watch out while hiring for the JavaScript coding interview process. Some of the red flags to avoid are mentioned below.
1. Lack of Problem-Solving Skills
This is a decision-maker because a lack of problem-solving skills shows the candidate is not skilled enough to perform the task. This includes a lack of time management or an inability to debug the task, such as struggling with identifying and fixing errors or rushing to conclusions without evaluating and solving the problem.
2. Poor Code Quality
Poor code writing quality includes coding that cannot be understood, maintained, or debugged. This can lead to performance issues and create problems in passing the interview.
3. Lack of Curiosity
Showing little or no interest or lack of interest in learning new things in the coding and programming language shows that the interviewee does not have an enthusiastic approach very much needed for the position.
4. Negative Attitude
Negative attitudes, such as complaining about the problem, do not solve the issues caused. Lack of interest or enthusiasm also portrays a negative attitude towards the job.
Remember that the coding interview is about technical skills and an opportunity to showcase problem-solving skills, communication skills, and overall approach and attitude. By avoiding these red flags, candidates can increase their chances of landing the job and make an excellent first impression.
FAQs
1. What is NaN in JavaScript?
NaN stands for "Not a Number" and checks whether a value is valid. It's generated when a value cannot be computed, or a non-numeric value cannot be converted to a number.
2. What Is the Difference Between == And === In JavaScript?
== (loose equal) compares two variables regardless of their data type. === (strict equal) compares two variables and checks their data type.
3. What Is the Concept of Prototype Inheritance?
Prototype inheritance is a JavaScript feature that allows objects to inherit properties and methods from other objects.
Grow your business with factoHR today
Focus on the significant decision-making tasks, transfer all your common repetitive HR tasks to factoHR and see the things falling into their place.
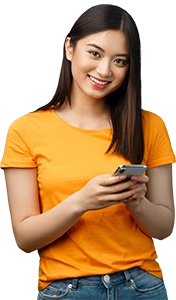
© 2024 Copyright factoHR